Understanding TailwindCSS
Understanding why tailwind exists and the benefits we get from using it/how powerful it is
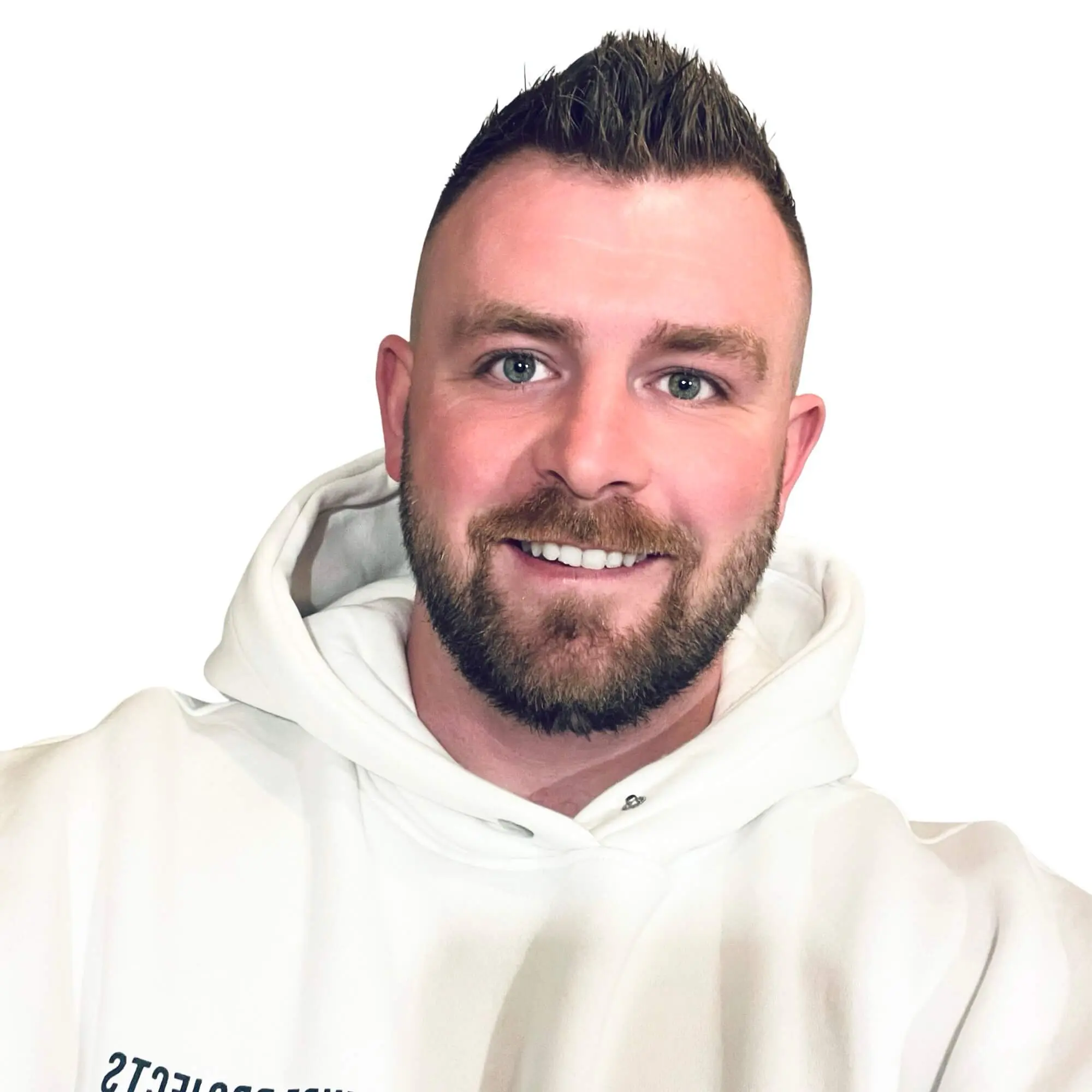
The most reusable components are those with class names that are independent of the content
Nicolas Gallagher - "About HTML semantics and front-end architecture"
Old style CSS/HTML semantics
With this approach we would define our HTML that we want (our content), apply a class name that is specifically coupled directly with this piece of code then write our corresponding CSS.
The problem with this is that, very quickly we find ourselves with a huge un-maintainable stylesheet with lots of class names for specific pieces of content that are directly related to unique use-cases. There are most likely duplicated classes for different unique use-cases as well as duplicated utilities baked into certain styles.
Example
Lets take this as an example;
I want a user profile section that looks like a card, it has rounded corners and breathing room with padding within the container. The background should be white and the text black and the container should have a shadow.
Our HTML markup would look something like this;
HTML
<div class="user-profile">
<img src="./path-to-image.png" />
<div>
<h2>Alan Montgomery</h2>
<p>Frontend Devleopment Lead</p>
</div>
</div>
Our CSS class would look something like this;
CSS
.user-profile {
background-color: white;
border-radius: 10px;
color: black;
padding: 2rem;
box-shadow: 0px 0px 10px 1px;
}
Now, imagine we wanted the exact same container for another piece of content for something like User Settings. Due to this approach and these types of semantics, we would need another class in our style-sheet for this;
.user-settings {
background-color: white;
border-radius: 10px;
color: black;
padding: 2rem;
box-shadow: 0px 0px 10px 1px;
}
You can see where I'm going with this. We would end up with a large amount of duplicated code for specific use-cases of content, containing very similar markup.
Abstracting CSS
One way we could improve this, if we take these very simple examples as a use-case, is look for what is common between these two containers' CSS. In this case, every property is the same, so lets abstract this and give it a class name of its own, independent of the content it's styling. With this, we could create another CSS class specifically for this like;
.card {
background-color: white;
border-radius: 10px;
color: black;
padding: 2rem;
box-shadow: 0px 0px 10px 1px;
}
If we take our user-profile
and user-settings
example HTML markup again and apply this, it would look like this;
<!-- User Profile -->
<div class="card">
<img src="./path-to-image.png" />
<div>
<h2>Alan Montgomery</h2>
<p>Frontend Devleopment Lead</p>
</div>
</div>
<!-- User Settings -->
<div class="card">
<div>
<h2>Privacy</h2>
<p>Manage privacy in this app</p>
</div>
</div>
See how our .card
CSS class has been re-used for both bits of content? This makes the CSS more maintainable, prevents duplication and means we could re-use this card
class anywhere we'd want in our website or application where we need a card of this style.
Further abstraction
Now, lets think about our little card
style. What if we wanted a different variation of this card? Maybe one that didn't have the shadow?
Yes, we could create another class for something like card-without-shadow
or something and duplicate everything in the card
class. However, then we are back to the original issue aren't we?
What if we just abstract the one property? This not only means that we can create our container or "card" with/without a shadow, but it also means we could easily apply a shadow to any element or piece of content regardless of what it means;
.shadow {
box-shadow: 0px 0px 10px 1px;
}
Now we could have two different variations of our card
element, one with a shadow, and one without a shadow, very easily by doing the following;
<div class="card shadow">...</div>
<div class="card">...</div>
Seems straight forward doesn't it?
TailwindCSS
This is where TailwindCSS comes in. Tailwind abstracts all CSS properties into utility style class names that we can use independent of the content we are trying to style.
For example, our user-profile
container would become;
<div class="bg-white text-black p-8 rounded shadow">
<img src="./path-to-image.png" />
<div>
<h2>Alan Montgomery</h2>
<p>Frontend Devleopment Lead</p>
</div>
</div>
In this simple example, we've applied the utility class names for the background, text, padding, box-shadow (rounded) and the shadow. This is completely independent of the content within this container.
All of a sudden, we can build very complex, customisable components or elements with utility class names that are de-coupled from their actual use-case, easy to read and maintainable. With this example, if we wanted a card that didn't have rounded edges, we'd just leave off the rounded utility class name.
TailwindCSS Documentation
You can check out the official TailwindCSS Documentation here. There is a utility class for every CSS property available. Allowing us to create reusable components that are independent of the content.
Using with React
Lets take a simple React component and how we could use our little example use-case to create a customisable card based on parameters (props) we pass to our component to control which Tailwind utility classes get added.
// By default, this Card component will be rounded and have a shadow (true)
export const Card = ({rounded = true, shadow = true, children}) => {
// Class names can be conditionally added within the className itself
// However, to understand better, I've written it like this
let cardClassNames = "bg-white text-black p-8";
rounded && cardClassNames += " rounded";
shadow && cardClassNames += " shadow";
return (
<div className={cardClassNames}>
{children}
</div>
);
}
In this example we could pass a true/false into the rounded
or shadow
prop to control whether or not to apply these relative class names which in turn would style the Card
component differently.
// Card component without the rounded corners and without the shadow
<Card rounded={false} shadow={false}>
<img src="./path-to-image.png" />
<div>
<h2>Alan Montgomery</h2>
<p>Frontend Devleopment Lead</p>
</div>
</Card>
// Card component without the shadow
<Card shadow={false}>
<img src="./path-to-image.png" />
<div>
<h2>Alan Montgomery</h2>
<p>Frontend Devleopment Lead</p>
</div>
</Card>
Hopefully this gives a clear understanding of why TailwindCSS exists and how powerful it is. Feel free to reach out for any questions/queries.