Understanding Server Actions
Exploring the power of Server Actions in Next.js and how they revolutionize server-side operations in React applications
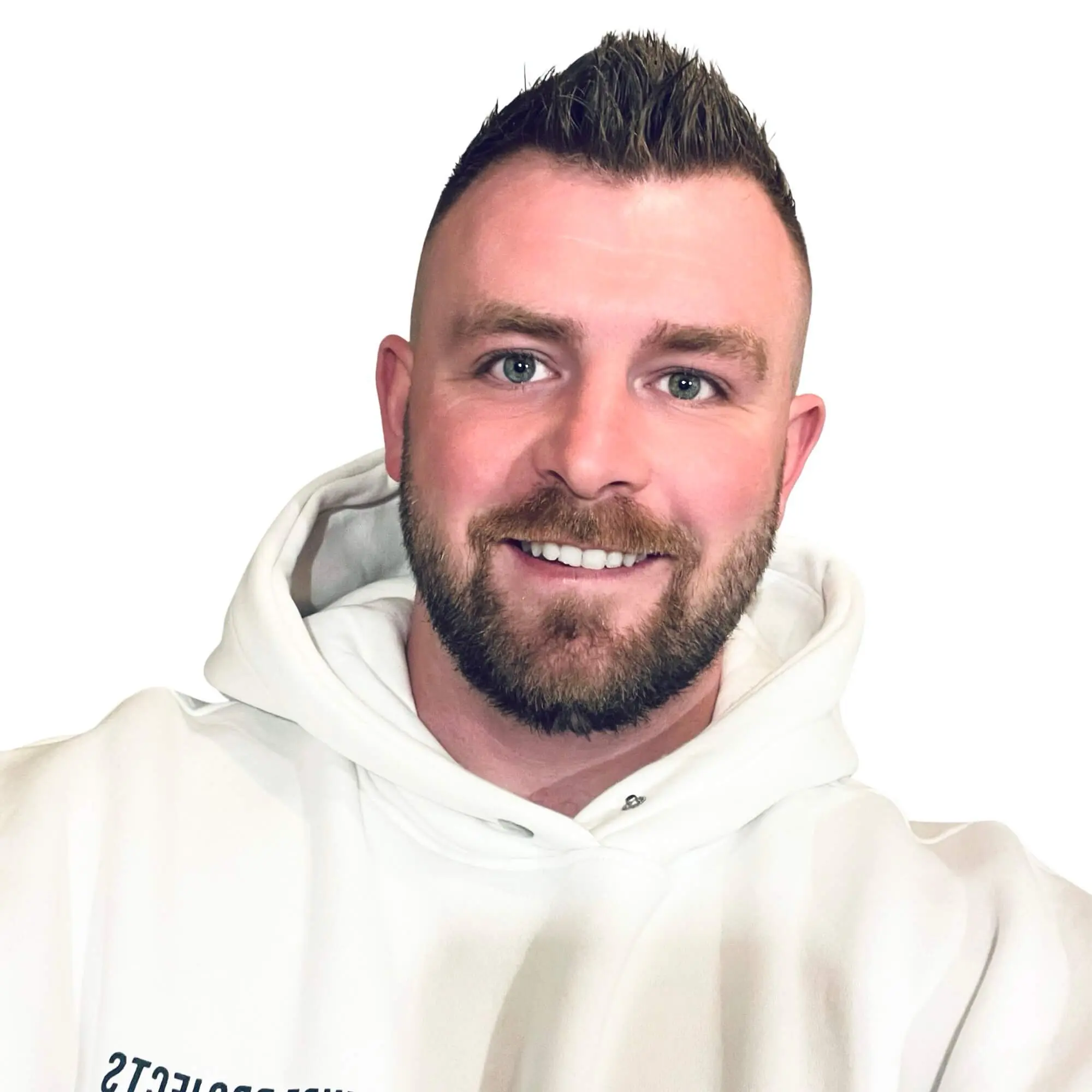
Server Actions are a powerful feature introduced in Next.js 13.4, allowing developers to define and execute server-side code directly within their React components. This seamless integration of server and client functionality opens up new possibilities for building efficient and interactive web applications.
What are Server Actions?
Server Actions are functions that run on the server but can be invoked from the client. They provide a way to perform server-side operations, such as database queries or API calls, without the need for separate API routes.
Let's look at a simple example:
"use server";
const addTodo = async (formData) => {
const todo = formData.get("todo");
// Add todo to database
await db.todos.create({ data: { text: todo } });
};
const TodoForm = () => {
return (
<form action={addTodo}>
<input type="text" name="todo" />
<button type="submit">Add Todo</button>
</form>
);
};
export default TodoForm;
In this example, addTodo
is a Server Action. It's defined with the 'use server'
directive, indicating that it should run on the server. The form's action
attribute is set to this function, allowing it to be called when the form is submitted.
Benefits of Server Actions
- Simplified Data Mutations: Perform database operations directly from your components without setting up API routes.
- Improved Security: Sensitive operations happen on the server, keeping your data safe.
- Reduced Client-Side JavaScript: Less code needs to be sent to the browser, improving performance.
- Progressive Enhancement: Forms work even without JavaScript enabled on the client.
Advanced Usage
Error Handling
Server Actions can throw errors, which can be caught and handled on the client:
"use server";
const submitForm = async (formData) => {
const email = formData.get("email");
if (!isValidEmail(email)) {
throw new Error("Invalid email address");
}
// Process form...
};
const Form = () => {
const [error, setError] = useState(null);
const handleSubmit = async (formData) => {
try {
await submitForm(formData);
} catch (e) {
setError(e.message);
}
};
return (
<form action={handleSubmit}>
<input type="email" name="email" />
<button type="submit">Submit</button>
{error && <p>{error}</p>}
</form>
);
};
export default Form;
Revalidation and Caching
Server Actions can be used to revalidate cached data:
"use server";
import { revalidatePath } from "next/cache";
const publishPost = async (formData) => {
const id = formData.get("id");
await db.post.update({ where: { id }, data: { published: true } });
revalidatePath("/blog");
};
const PublishButton = ({ postId }) => {
return (
<form action={publishPost}>
<input type="hidden" name="id" value={postId} />
<button type="submit">Publish</button>
</form>
);
};
export default PublishButton;
Combining with Client Components
Server Actions can be passed to client components, allowing for more dynamic interactions:
"use server";
const incrementLikes = async (id) => {
await db.post.update({ where: { id }, data: { likes: { increment: 1 } } });
};
const LikeButton = ({ postId }) => {
const [likes, setLikes] = useState(0);
const handleLike = async () => {
await incrementLikes(postId);
setLikes((prev) => prev + 1);
};
return <button onClick={handleLike}>Like ({likes})</button>;
};
export default LikeButton;
Streaming and Progressive Rendering
Server Actions can be combined with React's Suspense and streaming capabilities for progressive rendering:
"use server";
const loadComments = async (postId) => {
const comments = await db.comment.findMany({ where: { postId } });
return comments;
};
const Post = ({ id }) => {
return (
<article>
<h1>Post Title</h1>
<p>Post content...</p>
<Suspense fallback={<p>Loading comments...</p>}>
<Comments postId={id} loadComments={loadComments} />
</Suspense>
</article>
);
};
const Comments = ({ postId, loadComments }) => {
const comments = use(loadComments(postId));
return (
<ul>
{comments.map((comment) => (
<li key={comment.id}>{comment.text}</li>
))}
</ul>
);
};
export default Post;
Server-Only Modules
Next.js introduces the concept of server-only modules, which can be used with Server Actions to ensure certain code never reaches the client:
"use server";
import { encrypt } from "server-only-crypto";
const submitSensitiveData = async (formData) => {
const data = formData.get("sensitive");
const encrypted = encrypt(data);
// Store encrypted data...
};
Performance Considerations
When using Server Actions, consider the following performance optimizations:
-
Debouncing and Throttling: For frequently called actions, implement debouncing or throttling on the client side to reduce server load.
-
Optimistic Updates: Update the UI immediately before the server action completes, then reconcile with the server response:
"use client";
import { useState } from "react";
import { incrementLikes } from "./actions";
const LikeButton = ({ postId, initialLikes }) => {
const [likes, setLikes] = useState(initialLikes);
const handleLike = async () => {
setLikes((prev) => prev + 1); // Optimistic update
try {
await incrementLikes(postId);
} catch (error) {
setLikes((prev) => prev - 1); // Revert on error
console.error("Failed to increment likes:", error);
}
};
return <button onClick={handleLike}>Like ({likes})</button>;
};
export default LikeButton;
- Batching Actions: Combine multiple actions into a single server request when possible:
"use server";
const batchedActions = async (actions) => {
const results = await Promise.all(actions.map((action) => action()));
return results;
};
Security Considerations
While Server Actions provide improved security by keeping sensitive operations on the server, it's important to implement additional security measures:
- Input Validation: Always validate and sanitize input data on the server side:
"use server";
import { z } from "zod";
const UserSchema = z.object({
name: z.string().min(2).max(50),
email: z.string().email(),
age: z.number().int().positive().max(120),
});
const createUser = async (formData) => {
const userData = Object.fromEntries(formData);
try {
const validatedData = UserSchema.parse(userData);
// Proceed with user creation...
} catch (error) {
throw new Error("Invalid user data");
}
};
- Rate Limiting: Implement rate limiting to prevent abuse:
"use server";
import { rateLimit } from "./rate-limiter";
const submitComment = async (formData) => {
const userId = formData.get("userId");
await rateLimit(userId, "submit-comment", 5, "1m");
// Process comment submission...
};
- CSRF Protection: While Next.js provides built-in CSRF protection, ensure it's properly configured and consider additional measures for sensitive actions.
Testing Server Actions
Testing Server Actions requires a combination of unit tests and integration tests:
- Unit Testing: Test the logic of your Server Actions in isolation:
import { jest } from "@jest/globals";
import { incrementLikes } from "./actions";
import { db } from "./db";
jest.mock("./db");
test("incrementLikes increases likes count", async () => {
const mockUpdate = jest.fn();
db.post.update.mockResolvedValue({ likes: 1 });
await incrementLikes("post-1");
expect(db.post.update).toHaveBeenCalledWith({
where: { id: "post-1" },
data: { likes: { increment: 1 } },
});
});
- Integration Testing: Use tools like Playwright or Cypress to test the full flow, including client-side interactions:
test("like button increments likes", async ({ page }) => {
await page.goto("/post/1");
const likeButton = page.getByRole("button", { name: /like/i });
const initialLikes = await likeButton.innerText();
await likeButton.click();
await page.waitForTimeout(1000); // Wait for action to complete
const updatedLikes = await likeButton.innerText();
expect(parseInt(updatedLikes)).toBeGreaterThan(parseInt(initialLikes));
});
Conclusion
Server Actions in Next.js represent a significant leap forward in bridging the gap between server and client-side operations. By allowing developers to write server-side logic directly within their React components, Server Actions simplify data mutations, enhance security, and improve overall application architecture.
As you incorporate Server Actions into your Next.js projects, remember to:
- Keep your actions focused and modular
- Implement proper error handling and input validation
- Consider performance optimizations like debouncing and optimistic updates
- Maintain strong security practices
- Write comprehensive tests for both the actions themselves and their integration with the UI
Server Actions are still an evolving feature, so stay tuned to the official Next.js documentation and community resources for the latest best practices and updates. By mastering Server Actions, you'll be well-equipped to build more efficient, secure, and user-friendly web applications with Next.js.