Prettier Auto Formatting
Setting up Prettier for auto formatting
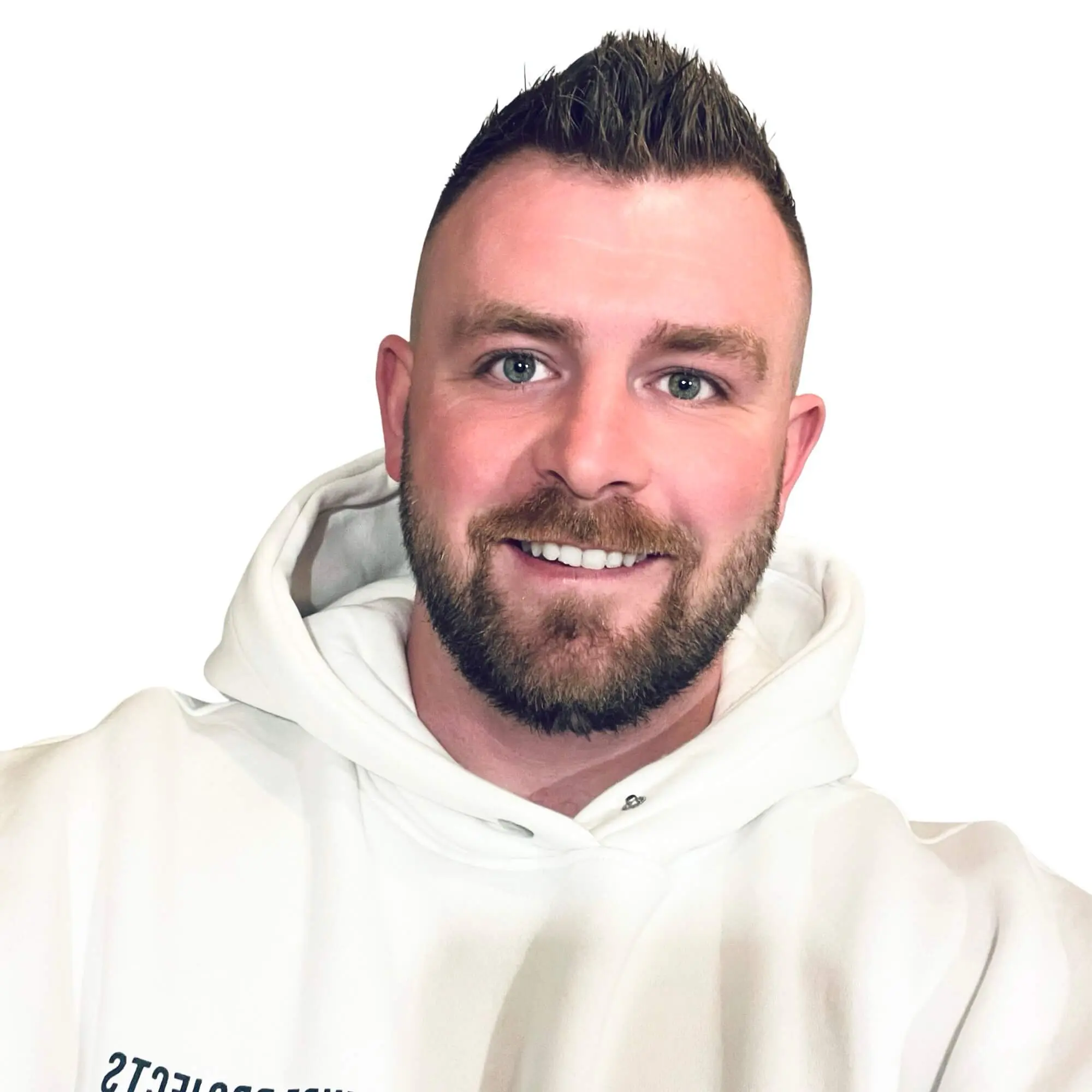
What is Prettier?
Prettier is an opinionated code formatter that automatically formats code according to a set of defined rules. It supports a wide range of programming languages and integrates seamlessly into various development environments. Its primary goal is to ensure code consistency and readability across your entire project by enforcing a uniform style.
Key Benefits of Using Prettier
Consistent Code Style
One of the most significant advantages of Prettier is its ability to enforce a consistent code style across your codebase. By using Prettier, you eliminate discrepancies in formatting that often arise from different team members having their own style preferences. This consistency improves code readability and maintainability.
Reduced Code Review Overhead
Prettier reduces the time spent on code reviews by formatting code automatically. Reviewers can focus on the logic and functionality of the code rather than debating style issues. This streamlined process helps accelerate the development workflow and ensures that feedback is more focused and constructive.
Enhanced Readability
Consistent formatting makes code easier to read and understand. Prettier enforces rules that improve the visual structure of the code, such as aligning brackets and maintaining proper indentation. This clarity helps developers quickly grasp the purpose and functionality of the code, reducing the likelihood of errors and misunderstandings.
Reduced Merge Conflicts
When multiple developers are working on the same codebase, inconsistent formatting can lead to frequent merge conflicts. Prettier minimizes these conflicts by ensuring that code is uniformly formatted before changes are committed. This approach reduces the time and effort required to resolve conflicts and merge code effectively.
Automated Formatting
Prettier integrates with various tools and editors, allowing you to automate code formatting as part of your development workflow. Whether you format code on save, paste, or by running a command, Prettier handles the task efficiently, freeing developers from manual formatting tasks and ensuring code adheres to project standards.
Support for Multiple Languages
Prettier supports a wide array of programming languages and file types, including JavaScript, TypeScript, HTML, CSS, Markdown, and more. This broad support allows teams to maintain a consistent style across different parts of their projects, regardless of the languages or technologies used.
Configurability
While Prettier is opinionated, it offers configuration options to tailor formatting rules to fit your project's needs. You can specify rules for line length, semicolons, quotes, and more. This flexibility ensures that Prettier can be adapted to align with your team's preferred coding practices while still providing the benefits of automated formatting.
Prettier is a valuable tool for modern development workflows, offering benefits that extend beyond mere code formatting. By enforcing consistent style, reducing review overhead, and automating formatting tasks, Prettier helps teams write cleaner, more maintainable code. Its integration with various tools and support for multiple languages make it an essential component of any development environment, streamlining the coding process and improving overall productivity.
Install the Prettier Package
You can install Prettier globally using npm. This makes Prettier available for use in any project:
npm install prettier -g
Alternatively, you can install Prettier as a development dependency within your project:
npm install --save-dev prettier
Ensure a Prettier Config Exists
For Prettier to work with specific formatting rules, you’ll need a configuration file. The configuration file is typically named .prettierrc. This file stores your project’s formatting preferences and ensures consistency across your codebase.
Create .prettierrc
In the root of your project, create a .prettierrc file with the following contents:
{
"arrowParens": "avoid",
"bracketSameLine": true,
"bracketSpacing": true,
"jsxSingleQuote": false,
"quoteProps": "consistent",
"semi": true,
"singleAttributePerLine": false,
"trailingComma": "none",
"singleQuote": false,
"printWidth": 200
}
This config defines various Prettier rules, such as:
arrowParens
: "avoid": Avoid parentheses for single-argument arrow functions.bracketSameLine
: true": Keep the closing bracket of a JSX element on the same line as the last prop.semi
: true: Always add a semicolon at the end of statements.trailingComma
: "none": No trailing commas for objects, arrays, etc.printWidth
: 200: Set a maximum line length of 200 characters.
Enable Formatting in VSCode
Prettier can be integrated with Visual Studio Code to format your files automatically when saving or pasting.
- Open VSCode, press CMD + SHIFT + P (on macOS) or CTRL + SHIFT + P (on Windows/Linux).
- Search for Preferences: Open Settings (JSON) and open it.
- Add the following configuration to your settings:
"editor.formatOnPaste": true,
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnType": true,
"files.autoSave": "off"
Explanation:
editor.formatOnPaste
: Automatically formats the pasted code.editor.formatOnSave
: Formats the file when you save it.editor.defaultFormatter
: Sets Prettier as the default formatter for JavaScript files.files.autoSave
: Disable auto-save if you’d rather save manually.
Running Prettier from the Command Line
You can also format your codebase directly from the command line, which is helpful for ensuring all files are correctly formatted before committing changes.
Adding an npm Script
- In your package.json, add the following script if it doesn’t already exist:
"scripts": {
"format": "prettier --write ."
}
- Now, run the following command in your terminal:
npm run format
This command will scan your entire project and format all the files based on your .prettierrc configuration.
By following these steps, you can set up Prettier to automatically format your code, ensuring consistency across your project. Prettier saves time by removing debates over code styling and improves readability by enforcing uniform standards.
Install the VSCode extension
There is a good Visual Studio Code Extension for Prettier which can be found here;
Husky and Pretty-Quick Integration
When Husky is enabled in your project, any staged files will be automatically formatted before the commit is finalized. This is achieved using pretty-quick
, which respects the configuration specified in your .prettierrc
file.
How It Works
Staged Files
Husky's pre-commit hook will trigger before a commit is completed. You can choose what runs here via the pre-commit
file found in /.husky
if it exists.
Automatic Formatting
pretty-quick
will run on the staged files to ensure they are formatted according to the rules defined in your .prettierrc
file.
Respecting Configuration
The formatting will adhere to the settings in the .prettierrc
file, ensuring consistency across your codebase.
This setup helps maintain code quality and uniformity in your project by automatically applying code formatting rules to the files being committed.